概要
これから数回にかけてREST APIについて学んだことを載せていく。
今回はREST APIの仕組みとプロジェクト作成方法について紹介する。
尚、RESTとは何かということについては取り扱わない。
仕組み
前提として、作成するREST APIアプリはJSON形式でクライアントと疎通を行うこととする。
クライアントサーバー間が疎通する全体像は以下のようになる。
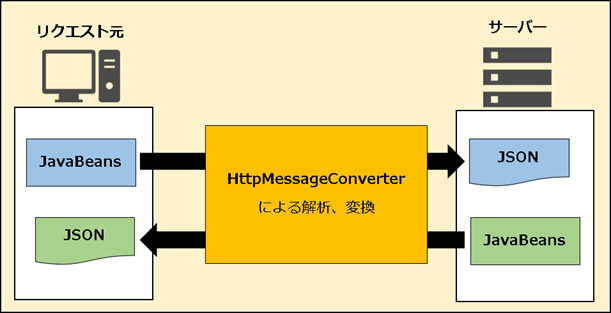
補足
サーバー(REST API)とリクエスト元(RestTemplateなど)を使用した疎通のポイントは以下となる。
クライアントとサーバー疎通
クライアントとサーバーは JSON 形式で疎通を行う。
クライアント側がJSONでリクエストを送信すると、サーバー側はそのJSONを解析してJavaBeansに変換する。
サーバー側がレスポンスを返す際には、JavaBeansをJSONに変換してクライアント側に送信する。
HttpMessageConverterの役割
JSONとJavaBeansの変換処理を担うのが、org.springframework.http.converter.HttpMessageConverterインターフェースとなる。
HttpMessageConverterは、リクエストのJSONをJavaオブジェクトに変換したり、レスポンスのJavaオブジェクトをJSONに変換する。
HttpMessageConverterの利用
HttpMessageConverterは、spring-web(spring-webmvcに内包)モジュールに含まれている。
HttpMessageConverterの実装クラスをカスタマイズすれば、返却するJSONを整形したりも可能。
今回は特にカスタマイズはしない。
プロジェクト作成
Spring MVCにてREST APIを作成するためのプロジェクト作成方法について紹介する。
基本的には以下のMavenセットアップの内容で作成は可能だが、不要な項目(jspなどのクライアント側の設定)があるため改めて記載する。
※Javaのバージョンは11を使用する
概要 Spring MVCフレームワークを使用して開発を進める際、Mavenプロジェクトの基本的なセットアップ方法についてまとめた。 前提 プロジェクトの具体的な作成方法については以下に記載。 [siteca[…]
Mavenプロジェクト作成
Springアプリに必要なライブラリを簡単にインストール管理できるMavenを使用してプロジェクトを作成する。
以下の流れで作成する。
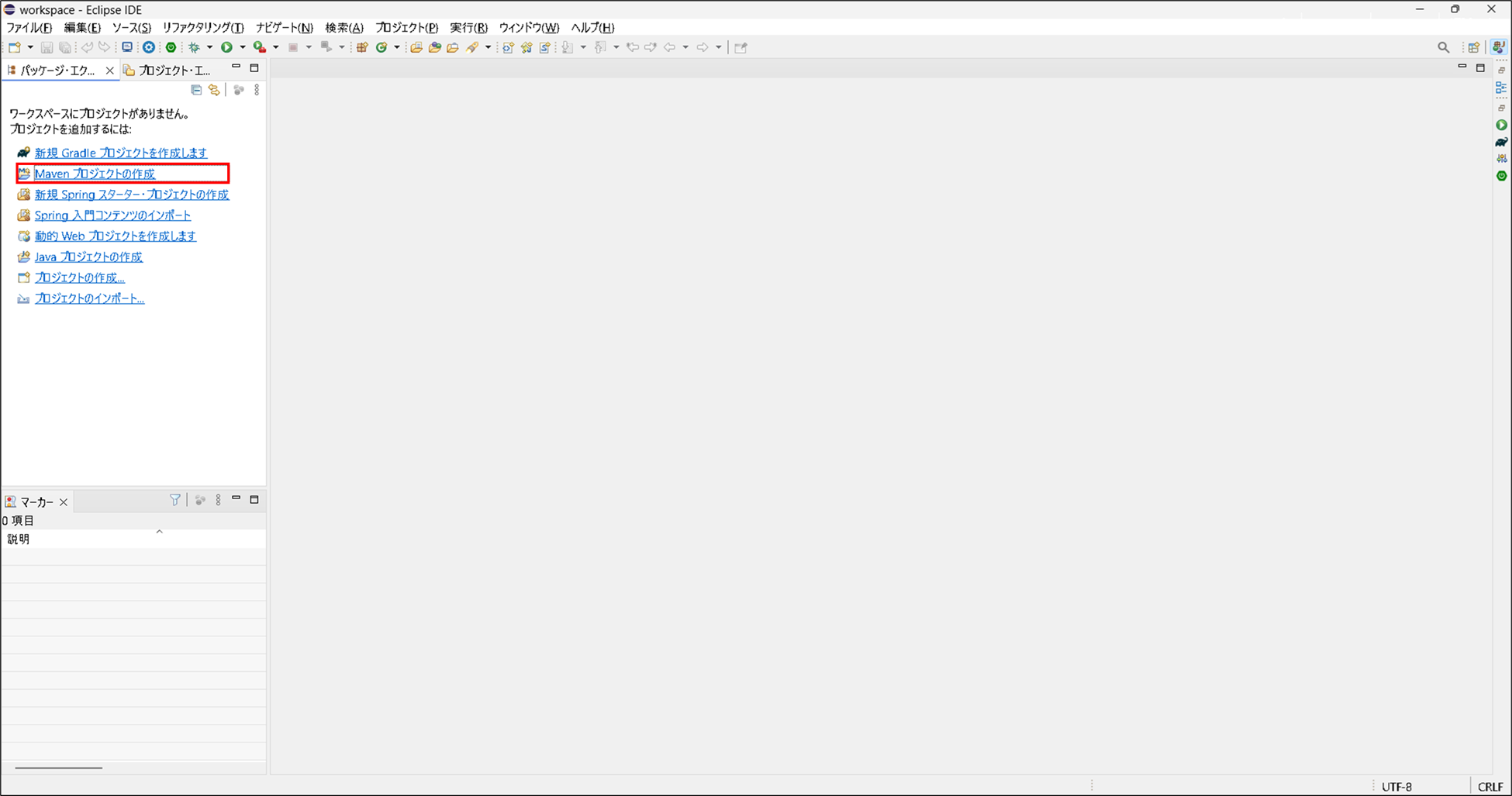
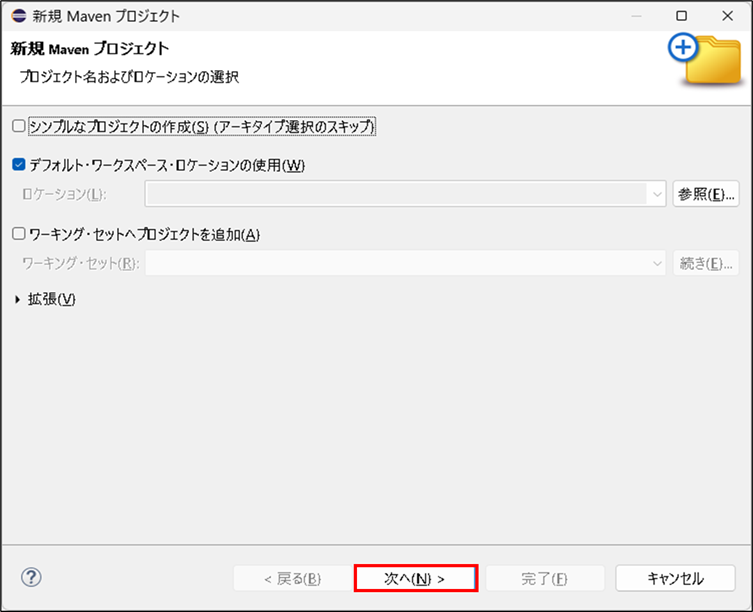
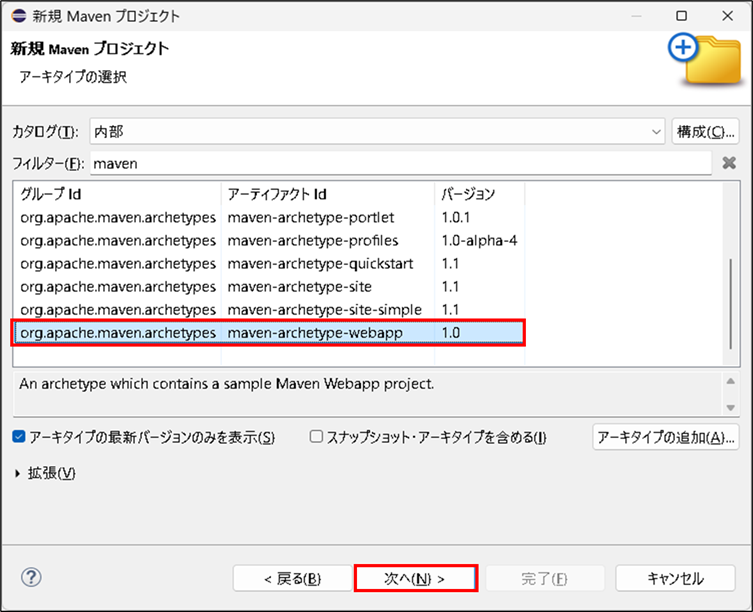
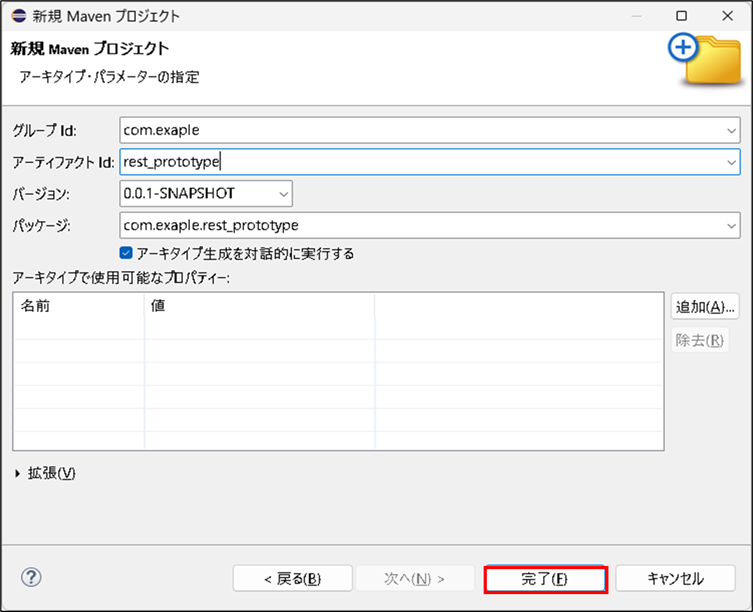
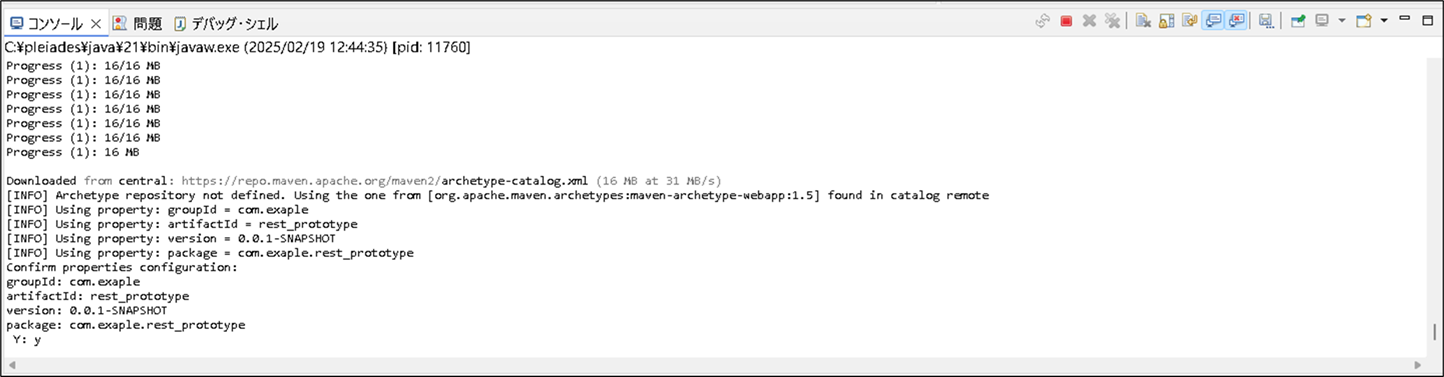
ファイル構成
準備するREST APIアプリの最終的なファイル構成は以下となる。
尚、今後必要に応じてファイル追加等は行っていく。
ファイル構成
pom.xml
以下のように設定する。(設定後は必要に応じてmaven installによりライブラリ資材をダウンロードする)
※今回はJavaのバージョンを11としている。
pom.xml
web.xml
ルートアプリケーションコンテキスト、Webアプリケーションコンテキストを初期化する。
以下のように設定する。
web.xml
logback.xml
必要に応じてファイルを作成する。
コンソールにログを表示させたい場合、以下のように設定する。
logback.xml
applicationContext.xml
ルートアプリケーションコンテキストが管理するBean定義ファイル。
以下のように設定する。
applicationContext.xml
springMVCContext.xml
Webアプリケーションコンテキストが管理するBean定義ファイル。
以下のように設定する。
springMVCContext.xml
Resource.java
クライアント側のURIリクエストに応じて返却するリソースオブジェクト。
以下のように定義している。
Resource.java
ResourceService.java
リソースの操作を行うビジネスロジッククラス。
DBを用意しない代わりに、マップ情報を定義している。
今後マップ操作を行うメソッドを追加していく。
ResourceService.java
Javaバージョンの設定
pom.xmlのJavaバージョンに合わせて、プロジェクトで使用するJavaのバージョンを設定する。
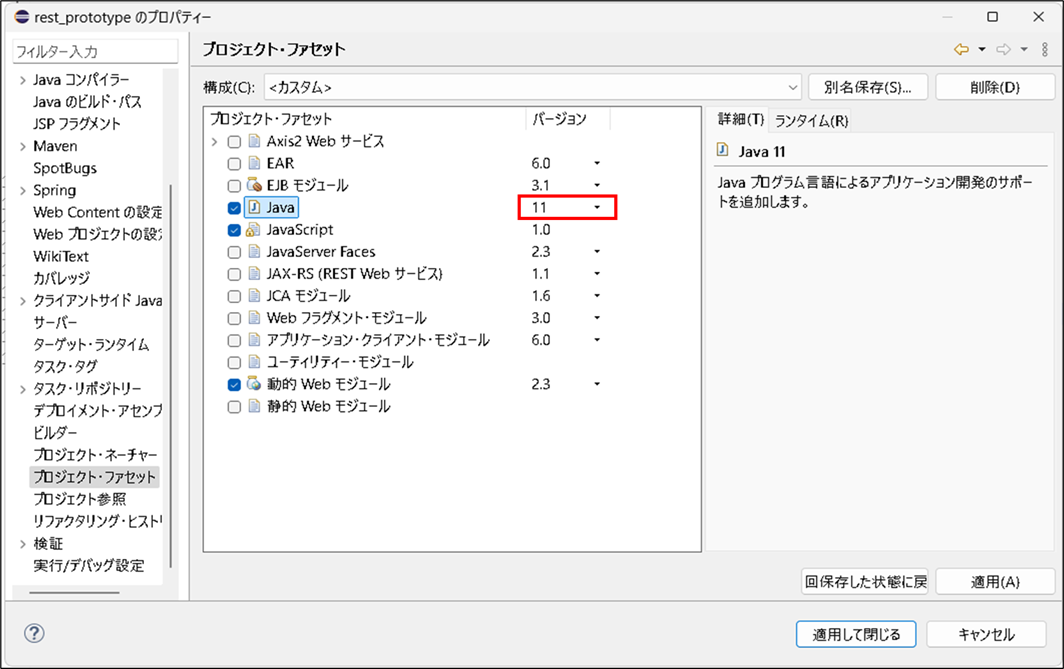
サーバー追加
作成したプロジェクトをTomcatに追加する。
以下の流れでサーバーを起動してエラーがなければ、REST APIアプリを作成するためのプロジェクトの準備は完了となる。
※Pleiadesに備え付けのTomcatで起動可能なTomcat9を使用した

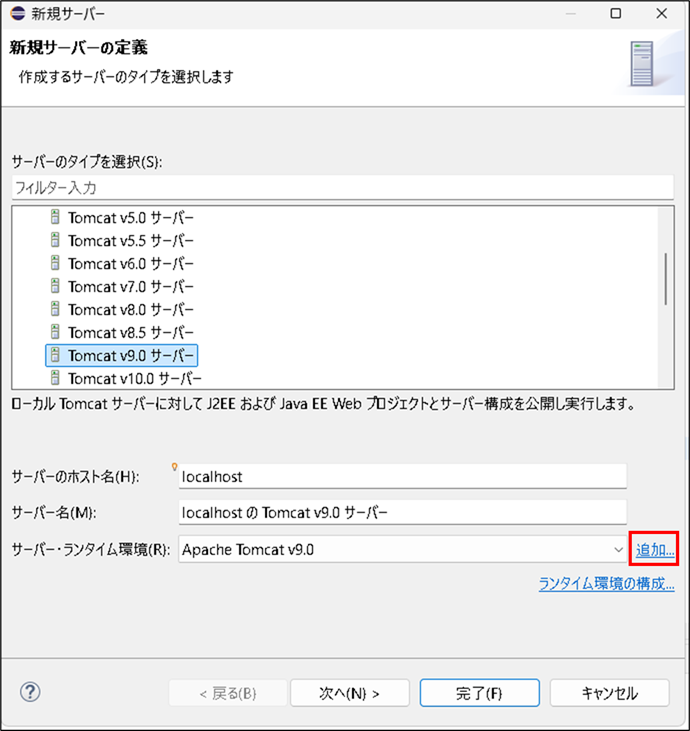
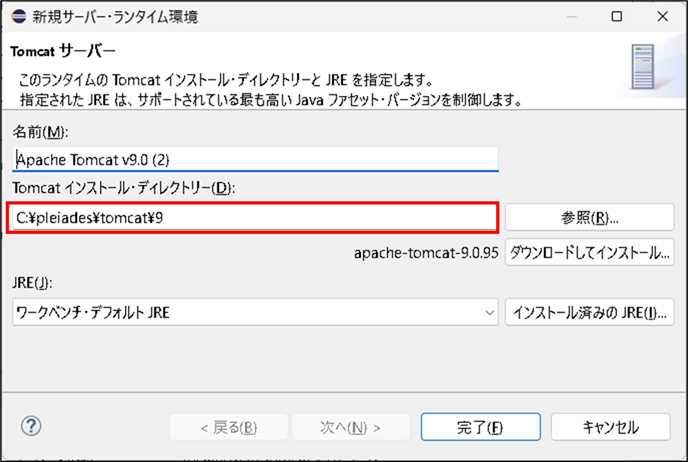
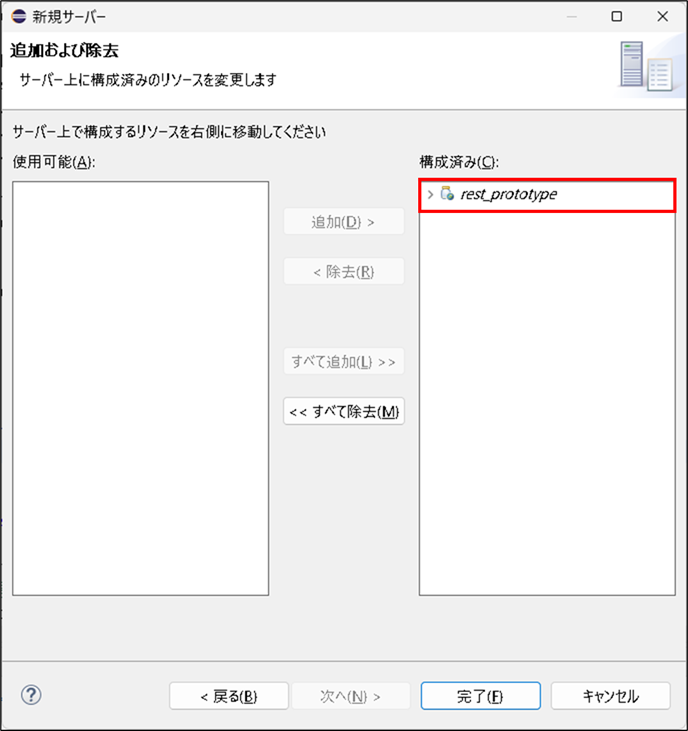
